For Loop Unity
Array
Let’s say you are creating a Beat Saber game, and need to keep track of when each block should appear during each music. We could create a variable for each block with the timestamp of its appearance, like so:
Esla, consider Unity's game loop as this: 1. For each script (sequentially), run its Update method. Update physics (apply velocity to each object's position, etc.) 3. Your method prevents Unity from moving on to step 2, so x can never change. Get code examples like 'unity for loop array' instantly right from your google search results with the Grepper Chrome Extension. In this article. This topic contains two examples that illustrate the Parallel.For method. The first uses the Parallel.For(Int64, Int64, Action) method overload, and the second uses the Parallel.For(Int32, Int32, Action) overload, the two simplest overloads of the Parallel.For method. You can use these two overloads of the Parallel.For method when you do not need to cancel the. Go to the animation and set it as looping.In the Animation window look at the bottom for something that should say 'Default' - it's a drop-down menu with looping options (Loop starts the animation over, PingPong plays it back and forth, Clamp Forever freezes the.
float block1 = 3.56f;
float block2 = 3.83f;
float block3 = 5.1f;
float block4 = 5.88f;
. . .
But that is simply impossible, especially with some music having over 1 thousand blocks. For these kinds of problems C# have Arrays, “containers” that store multiple data sequentially. So when you are dealing with enemies, quests, targets, etc, you will probably use arrays, or some similar data structure.
Strings are a special type of arrays, as they are a sequential collection of characters. But due to their frequent usage, C# provides a special built-in way to use them.
Building Arrays
As we said arrays store multiple data, but in order for the program, and us, understand how to access that data. For this, arrays follow some rules:
- Arrays can only store the same kind of data, that is, that share the same DataType.
- Array elements are stored sequentially, this way you find a specific data inside the structure.
// The following are arrays of ints, floats and booleans, respectively
int[] enemiesRemainingHealth;
float[] blockTimings;
bool[] enabledButtons;
You declare array variables much like any other variable, set a DataType, add the square brackets “[ ]” indicating this is an array, and finish it with the variable name. And just like any other variable, you can assign a value to it upon declaration, or re-assign a value later.
int[] enemiesRemainingHealth = { 100, 0, 58 } ;
float[] blockTimings = new float[] { 1.5f, 3.33f, 5.01f, 12, 12.12f };
bool[] enabledButtons;
enabledButtons = new bool[] { true, false };
// This will throw a compilation error. You may use this syntax only when declaring array variables
enemiesRemainingHealth = { 88, 15, 37, 84 };
The values stored in the array are declared inside the curly brackets “{ }”, they are added to the collection in the same order declared, and must fit the DataType of the array.
The new keyword will be explained in detail in the next chapter, for now think of it as if you were instantiating the array, “allocating space” for the whole “collection”.
Accessing Array Items
Like I said earlier, arrays store the data sequentially, and so, each entry can be accessed through an index:
// C# ignores white space, so sometimes declare an array
// in multiple lines, for better readability
string[] possibleAnswers = {
'I accept the quest!',
'Tell me why?!',
'Nah thanks'
};
// Prints 'Nah thanks' to the Unity Console
Debug.Log(possibleAnswers[2]);
In C# the arrays are indexed starting from 0, that is why the previous example prints “Nah thanks” instead of “Tell me Why?!” when using the index: 2.
Editing Arrays
Remember when I said the new keyword was to separate a space for the array? This means we can’t go changing an array size willy nilly. So if you want to alter the size of an array, refer back to the Building arrays section, and re-assign the array variable by creating a new array.
Changing the values stored in an array, on the other hand, is definitely possible! 🙂
// declares the playerIDs array variable, and create an array with 10 “slots” for string values
string[] playerIDs = new string[5];
playerIDs[0] = “BF49C0872A43EA4C5AA49AAC31709363A98E7105”;
int[] playerUnits = { 10, 10, 10, 10, 10 };
playerUnits[4] = 9;
playerUnits[1] += 2;
Using values inside an array is really similar to any other variable, just make sure to add which index of the array you are modifying and you are good to go!
When you declare an array and provides only its size, as we just did for “playerIDs”, each array entry is filled with the default value of the array DataType:
- int, float = 0
- bool = false;
- string = null;
Null values will also be covered in the next lesson. For now think of it like this: Remember that strings are arrays? When an array has a null value, it is because we still haven’t “allocated any space” for it, and so it is like it doesn’t exist.
Built-In Functionality
Arrays come with some built-in functionality for the most common operations done with arrays. I will cover the main ones, but you can find an extensive list at Microsoft documentation.
Array Length
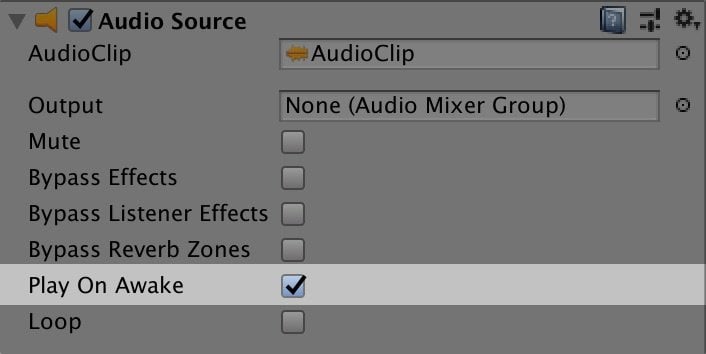
Knowing the size of an array is extremely useful, especially when declaring arrays with sizes that vary per execution.
int maxConcurrentPlayers = 3;
int[] currentPlayerIDs = new int[maxConcurrentPlayers];
// Prints 3 to the Unity Console
Debug.Log(currentPlayerIDs.Length);
Be careful not to try to access or modify values outside of the arrays bounds, doing so will give you an error: “IndexOutOfRangeException: Index was outside the bounds of the array.”
This is the Array.Length documentation for more info.
IndexOf Method
Next on the list is the Array.IndexOf method. This will search the provided array for a target value and return the index of the first occurrence, if there is one.
float[] spellCooldowns = { 3.17f, 0, 0, 33, -3.98f };
// Prints 3 to the Unity Console
Debug.Log(Array.IndexOf(spellCooldowns, 33));
// Prints 1 to the Unity Console, since it is the first occurrence.
Debug.Log(Array.IndexOf(spellCooldowns, 0));
// Prints -1 to the Unity Console, since it didn't find the target value
Debug.Log(Array.IndexOf(spellCooldowns, 999));
There are some overloads to this method, where you can provide a range for the search, make sure to check the documentation 🙂
Sort Method
Next up, Array.Sort! Oftentimes you need to set an array value in a specific order, for that we have the Sort method.

int[] playerScores = { 10, 80, 100, 30 };
// playerScores will be changed to { 10, 30, 80, 100 };
Array.Sort(playerScores);
Number arrays are sorted in ascending order (lowest to highest), while string arrays are sorted alphabetically. There are ways to change the sorting logic using overrides of this function, it uses more advanced techniques, but definitely take a look at the documentation if you ever need it!
Loops
So far we can store multiple data in arrays, but still, if we were to actually process that data, we still need to do it manually, one by one. And that is where loops come into play!
I won’t cover while and do..while loops here, because they are not used too much, and usually can be rewritten in terms of for and foreach loops.
For Loop
The for loop is used when you want to execute a block of code a X number of times. It follows the following syntax:
Unity Game Loop
for (initializer; condition; iterator)
{
// Block of code
}
Initializer
This is executed only once, before anything else, and it is mostly used to declare a variable that will be used during the iteration.
Condition
This statement must be a boolean expression. Before every loop, this expression is executed, if the condition evaluates to true, the loop keeps executing, otherwise it stops, and the control flow proceeds to the next statement after the for loop.
Iterator
The iterator statement executes after each loop, that is, after the condition and block of code are executed. It is most commonly used for incrementing the variable declared in the initializer.
int[] playerHealths = { 60, 10, 99 };
// This will create 3 messages on the Unity Console
// with the values 60, 10, 99, in this order.
for (int i = 0; i < playerHealths.Length; i++)
{
Debug.Log(playerHealths[i]);
}
It is a common practice to name the iterator variable “i” or “j” when “i” is already in use.
The iterator variable is only accessible inside the for block.
Tip: “i++” is equivalent to “i += 1”.
For Each Loop
Unity Foreach Loop
For each loop is a more readable way to write for loops. It is used when you don’t care about the values indexes, but only about the values themselves. It follows this syntax:
foreach (DataType variableName in collection)
{
// Block of code
}
The foreach will iterate in order over the entire collection provided, and on each iteration, set the variable “variableName” to the current value of the iteration. Make sure the DataType provided matches the DataType of the values inside the collection.
string[] commands = { “Grab”, “Release”, “Break” };
foreach (string command in commands)
{
// The variable “command” will be set to each of the values inside commands
Debug.Log(command);
}
For now you only know arrays, but foreach loops can be used with other collection structures as well, like Dictionaries and Lists, they use some more advanced features but are worth a look.
Coming up next!
Next week we’ll take a look at the heavily awaited Classes and Objects. With all the logic aspects out of the way, it’s time to see how it all fits together.
Happy coding 🙂
🔙 Back to the Learn C# for Unity menu
-->This topic contains two examples that illustrate the Parallel.For method. The first uses the Parallel.For(Int64, Int64, Action<Int64>) method overload, and the second uses the Parallel.For(Int32, Int32, Action<Int32>) overload, the two simplest overloads of the Parallel.For method. You can use these two overloads of the Parallel.For method when you do not need to cancel the loop, break out of the loop iterations, or maintain any thread-local state.
Note
This documentation uses lambda expressions to define delegates in TPL. If you are not familiar with lambda expressions in C# or Visual Basic, see Lambda Expressions in PLINQ and TPL.
The first example calculates the size of files in a single directory. The second computes the product of two matrices.
Directory size example
This example is a simple command-line utility that calculates the total size of files in a directory. It expects a single directory path as an argument, and reports the number and total size of the files in that directory. After verifying that the directory exists, it uses the Parallel.For method to enumerate the files in the directory and determine their file sizes. Each file size is then added to the totalSize
variable. Note that the addition is performed by calling the Interlocked.Add so that the addition is performed as an atomic operation. Otherwise, multiple tasks could try to update the totalSize
variable simultaneously.
Matrix and stopwatch example
This example uses the Parallel.For method to compute the product of two matrices. It also shows how to use the System.Diagnostics.Stopwatch class to compare the performance of a parallel loop with a non-parallel loop. Note that, because it can generate a large volume of output, the example allows output to be redirected to a file.
When parallelizing any code, including loops, one important goal is to utilize the processors as much as possible without over parallelizing to the point where the overhead for parallel processing negates any performance benefits. In this particular example, only the outer loop is parallelized because there is not very much work performed in the inner loop. The combination of a small amount of work and undesirable cache effects can result in performance degradation in nested parallel loops. Therefore, parallelizing the outer loop only is the best way to maximize the benefits of concurrency on most systems.


The Delegate
The third parameter of this overload of For is a delegate of type Action<int>
in C# or Action(Of Integer)
in Visual Basic. An Action
delegate, whether it has zero, one or sixteen type parameters, always returns void. In Visual Basic, the behavior of an Action
is defined with a Sub
. The example uses a lambda expression to create the delegate, but you can create the delegate in other ways as well. For more information, see Lambda Expressions in PLINQ and TPL.
The Iteration Value
The delegate takes a single input parameter whose value is the current iteration. This iteration value is supplied by the runtime and its starting value is the index of the first element on the segment (partition) of the source that is being processed on the current thread.
For Loop In Unity
If you require more control over the concurrency level, use one of the overloads that takes a System.Threading.Tasks.ParallelOptions input parameter, such as: Parallel.For(Int32, Int32, ParallelOptions, Action<Int32,ParallelLoopState>).
Return Value and Exception Handling
For returns a System.Threading.Tasks.ParallelLoopResult object when all threads have completed. This return value is useful when you are stopping or breaking loop iteration manually, because the ParallelLoopResult stores information such as the last iteration that ran to completion. If one or more exceptions occur on one of the threads, a System.AggregateException will be thrown.
In the code in this example, the return value of For is not used.
Analysis and Performance
For Loop Unity
You can use the Performance Wizard to view CPU usage on your computer. As an experiment, increase the number of columns and rows in the matrices. The larger the matrices, the greater the performance difference between the parallel and sequential versions of the computation. When the matrix is small, the sequential version will run faster because of the overhead in setting up the parallel loop.
Synchronous calls to shared resources, like the Console or the File System, will significantly degrade the performance of a parallel loop. When measuring performance, try to avoid calls such as Console.WriteLine within the loop.
Compile the Code
For Loop C# Unity
Copy and paste this code into a Visual Studio project.
See also
